· Diego Martin · tutorials · 4 min read
OpenAPI in .NET
Working with OpenAPI in .NET 9+, A step in the right direction
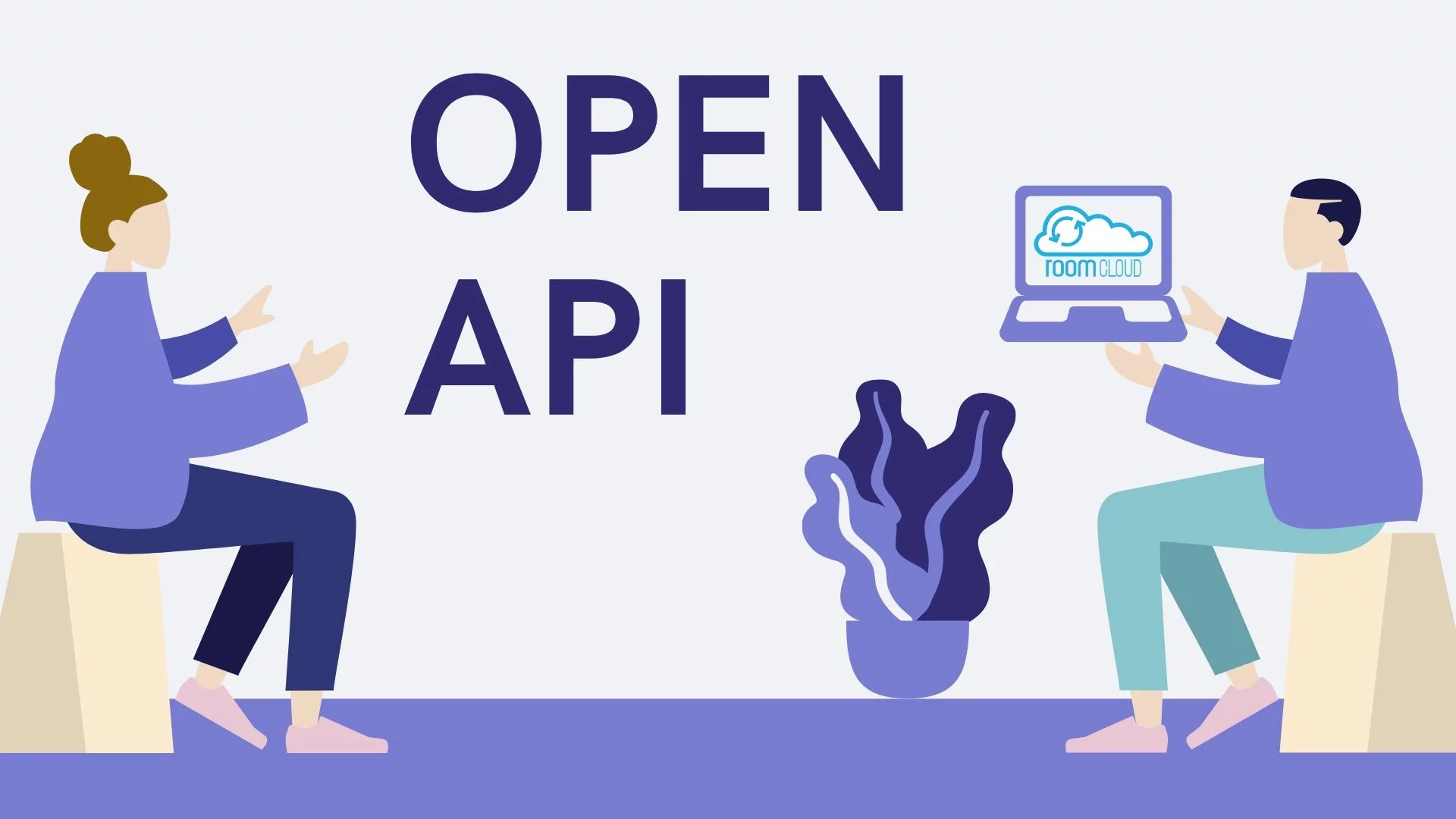
.NET 9 is bringing some exciting changes, especially around working with OpenAPI. If you’ve worked with OpenAPI before, you might know how painful it can be to set everything up. Historically, the integration in .NET has been tied to Swashbuckle, a popular NuGet package. However, starting with .NET 9, Microsoft is pushing things in a different direction by embracing their own package, making the experience smoother.
In this post, I’ll walk you through the setup of OpenAPI in .NET 9 using Microsoft’s new OpenAPI library. I’ll also cover generating OpenAPI JSON at build time, along with a preferred UI for visualization.
Let’s dive into a sample project and break it down step by step.
Setting up the Project
Create a basic .NET empty web project targeting .NET 9
var builder = WebApplication.CreateBuilder(args);
var app = builder.Build();
app.MapGet("/", () => "Hello World!");
app.Run();
Find all the code at my GitHub repository.
Endpoint to View OpenApi Document
To enable OpenAPI in your .NET 9 project, you need to install the Microsoft.AspNetCore.OpenApi
NuGet package. This package provides the necessary services to generate OpenAPI documentation for your API.
Once the package is installed, you can register the OpenAPI services with the following line:
builder.Services.AddOpenApi();
Add the OpenApi endpoint with the following line:
app.MapOpenApi();
Notice this is not a middleware but an endpoint.
With this configuration, when you run the application and navigate to /openapi/v1.json
, the OpenAPI JSON schema for your API will be served.
This JSON file is crucial for API consumers and for integration with other tools, such as testing clients or automatic documentation generators.
Build-Time OpenApi Generation
One of the most exciting features in .NET 9 is the ability to generate OpenAPI JSON at build time. This allows the OpenAPI schema to be available even before running the application, making it perfect for CI/CD pipelines.
To enable this feature, install the Microsoft.Extensions.ApiDescription.Server
NuGet package. This package introduces a MSBuild task behind the scenes that execute the application at build time to generate the OpenAPI schema.
After installing the package, when you build your project, the OpenAPI schema will be generated and stored in the obj
folder under a file named {ProjectName}.json
. For example, if your project is named WebApiOne
, the generated file will be located at obj/WebApiOne.json
This file can be utilized in CI/CD pipelines to ensure that your OpenAPI schema is always up-to-date and available for other processes like automated tests, documentation, or deployment configurations.
OpenApi UI
While Microsoft is providing a robust way to generate OpenAPI JSON, they are not planning to provide a UI for visualizing this schema. However, this isn’t necessarily a drawback. You have some popular options for displaying your API documentation, including:
Swagger UI: The most widely used OpenAPI UI tool. It provides an interactive way to explore your API endpoints and test them directly from the browser.
Scalar: Scalar provides a cleaner, more modern interface for OpenAPI visualization. I personally prefer Scalar for its simplicity and aesthetics.
Integrating Scalar
To integrate Scalar into your project, you need to install the Scalar.AspNetCore
package. This package adds the necessary UI endpoints for viewing your OpenAPI documentation.
After installing the package, you can expose the Scalar UI in development mode with:
app.MapScalarApiReference();
This will allow you to visualize your API documentation and try out the API directly from the browser by navigating to /scalar/v1.json
.
When testing with Scalar, you might run into cross-origin issues if your API and the Scalar UI are served from different origins. This is where the CORS configuration comes in.
builder.Services.AddCors(options =>
options.AddPolicy(
"AllowAll",
policy => policy
.AllowAnyOrigin()
.AllowAnyMethod()
.AllowAnyHeader()));
//...
app.UseCors("AllowAll");
This configuration ensures that the Scalar UI can interact with your API without running into cross-origin resource sharing issues. This is particularly useful during development, where you’re frequently testing and debugging your API endpoints.
Final Thoughts
With .NET 9, Microsoft is making significant strides towards improving the experience of working with OpenAPI. By embracing their own package for OpenAPI generation and making it easy to generate schemas at build time, they are simplifying the process for developers. The flexibility to integrate your preferred UI tool, like Scalar or Swagger UI, adds further value.